In 2011, the Microsoft Build developer conference rocked the Windows developer world by pitching JavaScript and HTML as the preferred way to develop applications for Windows 8. It was seen as a betrayal by many loyal .NET developers, and there was a lot of skepticism about Microsoft being able to lure web developers to develop for the Windows Store. At the time we were also introduced to UWP with much less fanfare, and I started to learn UWP because it was familiar to me as a Windows Phone and WPF developer.
This shift in Windows development got me thinking that if we could use HTML for the UI, and .NET for the logic, that I would feel more comfortable. JavaScript has come a long way since then, but at the time I didn’t want to get anywhere near it.
Introduction to Blazor
Now a decade later, thanks to advancements in web technologies and a desire to get native performance in the browser without the security concerns of ActiveX, this dream is becoming a reality with Blazor. Blazor leverages Razor templates to develop web apps using .NET and HTML. There are currently two options to develop Blazor apps. You can develop server side using SignalR under the covers to handle interaction with the UI. Alternatively, you can develop client side using WebAssembly and run it all inside the browser. A standalone version of .NET runs inside WebAssembly and the Blazor app runs on top of this. Real .NET running natively in the browser and no transpiling to JavaScript. Blazor also provides access to JavaScript, so a hybrid app could be made as needed. Blazor and WebAssembly in general are considered secure in the browser since the browser limits access to the underlying operating system.
We will focus on Blazor apps that run in WebAssembly, since this is what is most relevant to .NET MAUI. In Visual Studio 2022, there is an application template to create a Blazor WebAssembly App.
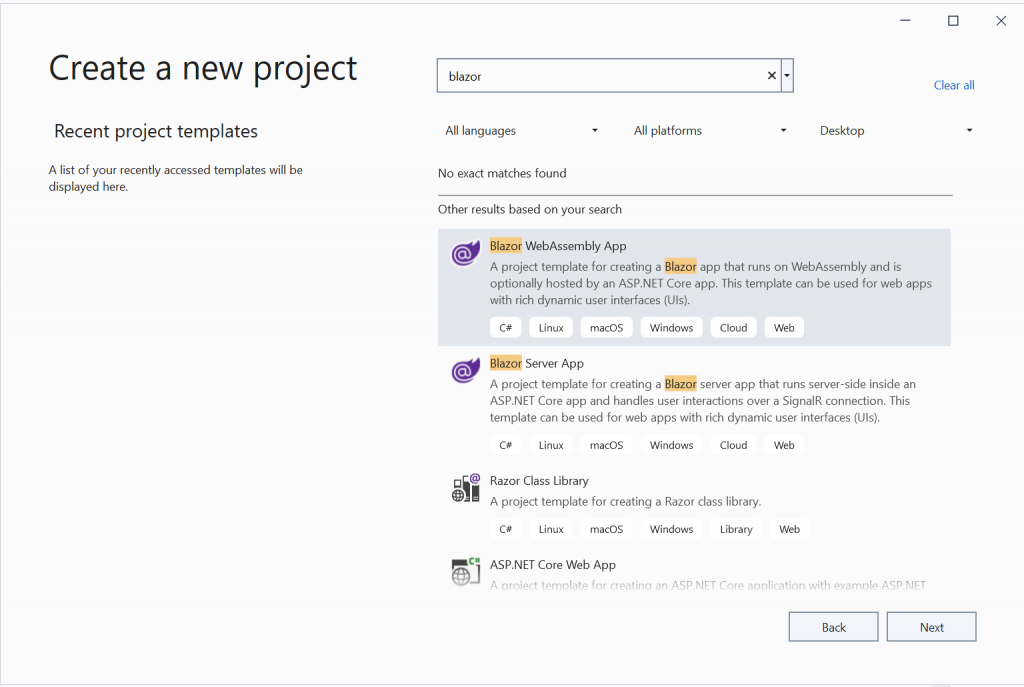
The project this creates has some basic functionality with a handful of sample Razor templates, and here is the app running:
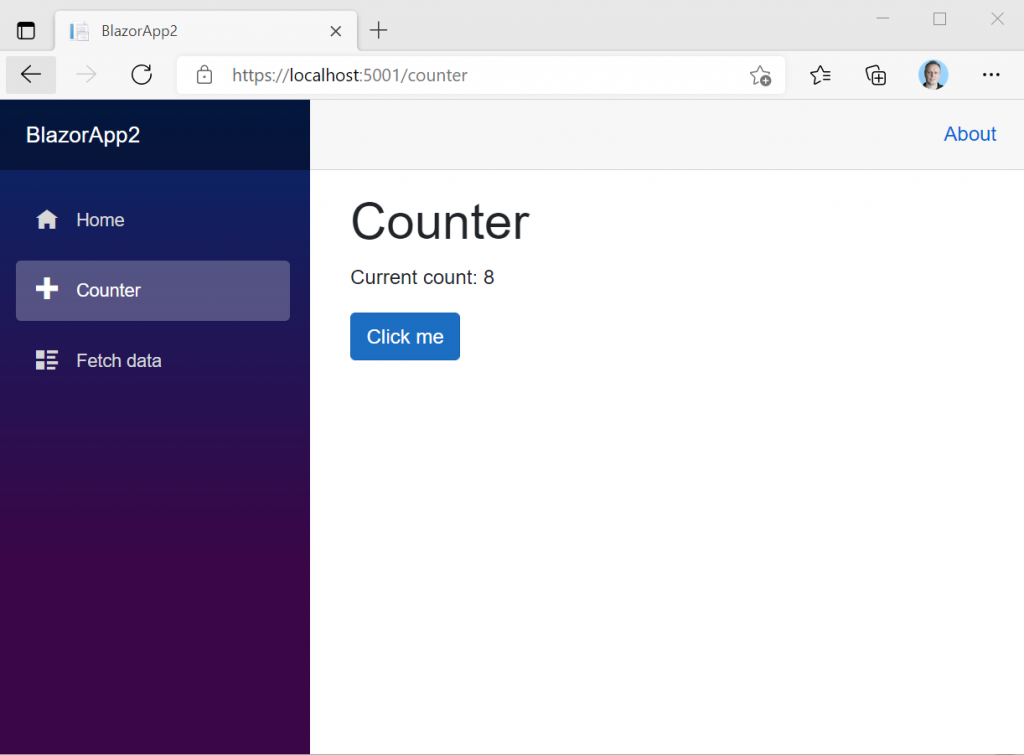
And here is the template for the counter tab:
@page "/counter"
<h1>Counter</h1>
<p role="status">Current count: @currentCount</p>
<button class="btn btn-primary" @onclick="IncrementCount">Click me</button>
@code {
private int currentCount = 0;
private void IncrementCount()
{
currentCount++;
}
}
Note the C# code block to handle the button click.
Blazor in MAUI
A Blazor app can also be packaged as a Progressive Web App (PWA), Electron app, or other web app technologies. The newest option is to run Blazor inside a .NET MAUI app. Now why is this interesting and what makes it appealing over these other techniques?
- Access to the entire .NET Framework. When Blazor web views run inside MAUI, they are not using WebAssembly or its own copy of .NET. The Blazor code actually runs in process with the MAUI app, and the Blazor code has access to everything that MAUI has access to, including platform APIs.
- Mix and match MAUI controls with Blazor web views as needed. Choose the right framework for the desired UI and mix and match, with the ability to have multiple Blazor web views on a single page if desired.
- Take advantage of the MAUI packaging experience, targeting the most popular platforms as a native app in the app store.
Since the release of .NET 6 Preview 4, this functionality has been available in MAUI and you can try it today. Again, like in a previous post, the tooling isn’t all integrated into Visual Studio yet, but you can create a Blazor Maui app as follows:
dotnet new maui-blazor -o MauiBlazorApp
Running this app on the desktop, it looks like this:
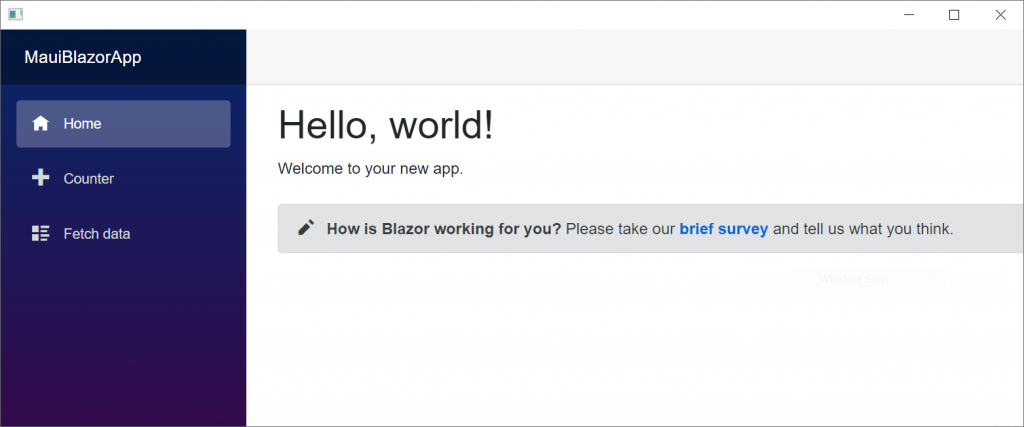
And here is the MainPage.xaml with the embedded BlazorWebView:
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:b="clr-namespace:Microsoft.AspNetCore.Components.WebView.Maui;assembly=Microsoft.AspNetCore.Components.WebView.Maui"
xmlns:local="clr-namespace:MauiBlazorApp"
x:Class="MauiBlazorApp.MainPage"
BackgroundColor="{DynamicResource PageBackgroundColor}">
<b:BlazorWebView HostPage="wwwroot/index.html">
<b:BlazorWebView.RootComponents>
<b:RootComponent Selector="app" ComponentType="{x:Type local:Main}" />
</b:BlazorWebView.RootComponents>
</b:BlazorWebView>
</ContentPage>
As mentioned earlier, you can embed multiple BlazorWebView controls as needed, and also add MAUI controls to the page.
I’m very excited to see the very creative uses that developers come up with to leverage Blazor inside MAUI. I personally have some of my own ideas that I’m interested to try out.